Introduction
In last week’s blog we discussed PHP as a server-side scripting language, we also got through the basics of PHP such as arrays. In this week’s blog entry we will focus on some of the more advanced mechanisms of php.
The HTTP is a stateless protocol which implies that a web server doesn’t differentiate or keep track of requests coming from a user’s browser. The hypertext transfer protocol doesn’t provide a way by which requests from different clients can be identified. Having a state-less systems means that no resources are taken to keep track of a requests state. Not being able to identify the client meant that a web application wasn’t able to know whether a user is logged in or what the user’s preferences were. This problem was countered by creating a mechanism called cookies by which states could be managed. To better understand cookies one should take a closer look at an instance of an HTTP transaction in conjunction with the cookie mechanism.

Whenever a user makes a request the server includes a Set-Cookie header in the response. This means that a cookie will be set and be available in future requests within the Cookie header. A cookie is stored on the client and data is stored in the form of a key-value pair. The cookie can be used to differentiate between clients. In PHP cookies are created by calling the SetCookie() function. The SetCookie() function must be placed before the HTML tag to have effect. The SetCookie() function takes a name which is used as a key to the value passes as a second parameter. Other arguments include the expiration date, path and the domain name. To get a value present in a cookie the $_COOKIE variable is used. A value stored in a $_COOKIE can be retrieved by allocating a key with the array notation [] in PHP such as $_COOKIE[“UserId”]. Deletion of a cookie is performed by setting the expiration date of a cookie in the past.
Sessions are used to extend state management over the web by storing data pertaining to a particular client. As opposed to cookies sessions are stored on the server while cookies are stored on the client’s machine. Data in sessions is stored in a session data store were it gets updated with each request. The unique id which identifies a record within a session data store is called the session identifier. In PHP the function call session_start() is called to determine whether a session identifier is included in the request. If a session is present then the data for that request is made avaiable in the $_SESSION variable. Retrieving sessions in PHP is similar to retrieving cookie except $_SESSION is used. To free a session variable the unset() function can be used while to completely destroy a session as the name implies the session_destroy() is used.
Task Summary
Throughout this section of this blog entry the following tasks will be performed;
Create login screen that accepts a user name and a password and which are validated on the server-side
A login screen is essentially a form from which a user can be authenticated to access some restricted section of a web site. In our current scenario the user will be authenticated to view a home page. The first thing I considered is the file I needed to create to have a good working environment. For this task I created three files; the login page (login.php), the login code behind file (logincb.php), a functions file and the home page.

Each of the files will contain code specific to the task at hand. In this case the user will navigate to the login screen through the portal. The login screen contains a text field for the user name a text field for the password and a submit button to post the page.

The controls mentioned are placed with a form tag which point to to the code behind file logincb.php. This means that a post action will be in effect on logincb.php on submitting the form. Once in the code behind file I started to validate the user inputted fields. The validation function will do the following tasks;
In PHP one can check if a field is empty by using an inbuilt function called empty() (Other functions such as isset() can be used. The main difference between empty() and isset() is that isset() return false whenever a variable doesn’t exist) which will return true if the fields are kept empty.

Each if statement check whether both user name and password are present. If one of the fields is missing the user is redirected to the login.php screen along with three arguments; the first argument holds the error message and the last two arguments determine which of the fields is empty and show an asterix next to the respective text field.

If the the fist part of this validation procedure is skipped the next step would be to validate the entered user name and password against a list of user names and passwords. The aforementioned list is created in a text file (cred.txt). The functions which will handle this part of the validation is hosted in the functions.php file. In order to gain access to the function the include() had to be used. The validateUserId() function creates a pointer to the text file by calling the fopen() function. Iterating through the list is accomplished by using a while loop which with each iteration checks for the end of file. Also with each iteration the line loaded from the text file is split using preg_split() function and compared to the user credentials entered by the user.
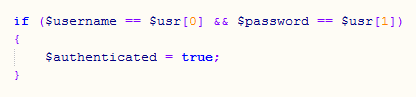
If details do not match any of the lines loaded an error message is return which is then obtained by using the $_GET variable and printing the message.

If the details entered match one of the lines loaded a true value is returned from the function and the user is redirected to the home page using the header(“Location:xxxxx”) construct. This concludes the second step of this validation algorithm.

Add a “remember me“ button that uses a cookie to remember a successful login.
The “remember me” feature is quite common amongst web sites. This feature allows for a cookie variable to be stored on the client’s machine. Whenever the client tries to navigate to the same page the web site will use the cookie stored to authenticate the user. The first thing included in the login screen was a check box with an name of “chkRemember”. On submit of the login screen whenever the checkbox is checked a cookie is set by using the setcookie() function with a duration of one week.

The user is then redirected to the home page. In the home page the user name is shown by accessing the cookie variable $_COOKIE. A post conditional statement was set to display the user name on the home page upon determining the way the home page was accessed.

An anchor tag is also included which provides the user a way to log out and expire the cookie. The anchor tag points to the code behind file logincb.php and passes a parameter logout=true which is then obtained by accessing the $_GET variable. A function was created in the functions.php file which expires a cookie given two parameters; the cookie name and the cookie value.

If the user decides to close the web page without logging out and tries to access the login page the user is redirected to the home page.

Using Sessions
Sessions extend the cookie concept on the web. By using sessions information can be transfered from one page to another. In this case the user name once authenticated will be displayed in each page by accessing the $_SESSION variable. Upon authentication the function session_start() is called. This function either starts or resumes a session based on the current session identifier. The session identifier can be obtained by calling the built-in function session_id(). After initialising the session the already authenticated details are assigned to the $_SESSION variable each with their respective names.

The user name is displayed in a place holder in the home.php page.

Each page that is visited after logging is started with the snippet shown below. This allows for the page to check if the user is logged in and if so to display the user name.

Conclusion
There are a number of security issues that one must watch out for when using cookies and sessions. One must be diligent by not storing sensitive information in cookies such as passwords.
In last week’s blog we discussed PHP as a server-side scripting language, we also got through the basics of PHP such as arrays. In this week’s blog entry we will focus on some of the more advanced mechanisms of php.
The HTTP is a stateless protocol which implies that a web server doesn’t differentiate or keep track of requests coming from a user’s browser. The hypertext transfer protocol doesn’t provide a way by which requests from different clients can be identified. Having a state-less systems means that no resources are taken to keep track of a requests state. Not being able to identify the client meant that a web application wasn’t able to know whether a user is logged in or what the user’s preferences were. This problem was countered by creating a mechanism called cookies by which states could be managed. To better understand cookies one should take a closer look at an instance of an HTTP transaction in conjunction with the cookie mechanism.
Whenever a user makes a request the server includes a Set-Cookie header in the response. This means that a cookie will be set and be available in future requests within the Cookie header. A cookie is stored on the client and data is stored in the form of a key-value pair. The cookie can be used to differentiate between clients. In PHP cookies are created by calling the SetCookie() function. The SetCookie() function must be placed before the HTML tag to have effect. The SetCookie() function takes a name which is used as a key to the value passes as a second parameter. Other arguments include the expiration date, path and the domain name. To get a value present in a cookie the $_COOKIE variable is used. A value stored in a $_COOKIE can be retrieved by allocating a key with the array notation [] in PHP such as $_COOKIE[“UserId”]. Deletion of a cookie is performed by setting the expiration date of a cookie in the past.
Sessions are used to extend state management over the web by storing data pertaining to a particular client. As opposed to cookies sessions are stored on the server while cookies are stored on the client’s machine. Data in sessions is stored in a session data store were it gets updated with each request. The unique id which identifies a record within a session data store is called the session identifier. In PHP the function call session_start() is called to determine whether a session identifier is included in the request. If a session is present then the data for that request is made avaiable in the $_SESSION variable. Retrieving sessions in PHP is similar to retrieving cookie except $_SESSION is used. To free a session variable the unset() function can be used while to completely destroy a session as the name implies the session_destroy() is used.
Task Summary
Throughout this section of this blog entry the following tasks will be performed;
- Create a login screen that accepts a user name and a password which are validated on the server-side.
- Add a “remember me“ button that uses a cookie so that the user does not have to log in again.
- Use sessions to store user information
Create login screen that accepts a user name and a password and which are validated on the server-side
A login screen is essentially a form from which a user can be authenticated to access some restricted section of a web site. In our current scenario the user will be authenticated to view a home page. The first thing I considered is the file I needed to create to have a good working environment. For this task I created three files; the login page (login.php), the login code behind file (logincb.php), a functions file and the home page.
Each of the files will contain code specific to the task at hand. In this case the user will navigate to the login screen through the portal. The login screen contains a text field for the user name a text field for the password and a submit button to post the page.
The controls mentioned are placed with a form tag which point to to the code behind file logincb.php. This means that a post action will be in effect on logincb.php on submitting the form. Once in the code behind file I started to validate the user inputted fields. The validation function will do the following tasks;
- Check that required fields are not empty
- Validate the fields with the user name and password in store.
In PHP one can check if a field is empty by using an inbuilt function called empty() (Other functions such as isset() can be used. The main difference between empty() and isset() is that isset() return false whenever a variable doesn’t exist) which will return true if the fields are kept empty.
Each if statement check whether both user name and password are present. If one of the fields is missing the user is redirected to the login.php screen along with three arguments; the first argument holds the error message and the last two arguments determine which of the fields is empty and show an asterix next to the respective text field.
If the the fist part of this validation procedure is skipped the next step would be to validate the entered user name and password against a list of user names and passwords. The aforementioned list is created in a text file (cred.txt). The functions which will handle this part of the validation is hosted in the functions.php file. In order to gain access to the function the include() had to be used. The validateUserId() function creates a pointer to the text file by calling the fopen() function. Iterating through the list is accomplished by using a while loop which with each iteration checks for the end of file. Also with each iteration the line loaded from the text file is split using preg_split() function and compared to the user credentials entered by the user.
If details do not match any of the lines loaded an error message is return which is then obtained by using the $_GET variable and printing the message.
If the details entered match one of the lines loaded a true value is returned from the function and the user is redirected to the home page using the header(“Location:xxxxx”) construct. This concludes the second step of this validation algorithm.
Add a “remember me“ button that uses a cookie to remember a successful login.
The “remember me” feature is quite common amongst web sites. This feature allows for a cookie variable to be stored on the client’s machine. Whenever the client tries to navigate to the same page the web site will use the cookie stored to authenticate the user. The first thing included in the login screen was a check box with an name of “chkRemember”. On submit of the login screen whenever the checkbox is checked a cookie is set by using the setcookie() function with a duration of one week.
The user is then redirected to the home page. In the home page the user name is shown by accessing the cookie variable $_COOKIE. A post conditional statement was set to display the user name on the home page upon determining the way the home page was accessed.
An anchor tag is also included which provides the user a way to log out and expire the cookie. The anchor tag points to the code behind file logincb.php and passes a parameter logout=true which is then obtained by accessing the $_GET variable. A function was created in the functions.php file which expires a cookie given two parameters; the cookie name and the cookie value.
If the user decides to close the web page without logging out and tries to access the login page the user is redirected to the home page.
Using Sessions
Sessions extend the cookie concept on the web. By using sessions information can be transfered from one page to another. In this case the user name once authenticated will be displayed in each page by accessing the $_SESSION variable. Upon authentication the function session_start() is called. This function either starts or resumes a session based on the current session identifier. The session identifier can be obtained by calling the built-in function session_id(). After initialising the session the already authenticated details are assigned to the $_SESSION variable each with their respective names.
The user name is displayed in a place holder in the home.php page.
Each page that is visited after logging is started with the snippet shown below. This allows for the page to check if the user is logged in and if so to display the user name.
Conclusion
There are a number of security issues that one must watch out for when using cookies and sessions. One must be diligent by not storing sensitive information in cookies such as passwords.
No comments:
Post a Comment